General-purpose TMS Optimization¶
Note
When using this feature in a publication, please cite Worbs T, Rumi B, Madsen KH, Thielscher A, Personalized electric field simulations of deformable large TMS coils based on automatic position and shape optimization, bioRxiv 2024.12.27.629331
Introduction¶
For setting up a general-purpose, flexible TMS optimization, you need to select the head model and the coil. In addition, you have to indicate whether your aim is to place a coil smoothly on the head surface, or whether you want to optimize its placement to maximize the electric field magnitude in a region-of-interest (ROI) in the brain:
For the first case, you will have to provide a starting position on the head surface. The algorithm then adjusts this position and deforms the coil to smoothly fit the coil casing on the head surface, thereby ensuring a minimal distance to the head surface at all positions.
For the second case, you need to provide a target region-of-interest (ROI) in the brain.
In both cases, the coil position (and shape in case of flexible coils) will be chosen to prevent intersections of the coil casing with the head. The extent of position changes can be controlled by the user by the means of (pre-)defined translation and rotation ranges.
Example 1: Optimizing the electric field magnitude in a ROI¶
The following example optimizes the postion of a figure-8 coil to maximize the electric magnitude in a ROI around the left hand knob. As standard, a distance between the coil casing and head of 4 mm is ensured (as rough estimate of the distance caused by the hair). Here, it is set to 0 mm as example.
Python
''' Basic example demonstrating optimization of the electric field strength in region of interest for a flat figure-8 coil The coil center will be placed to maximize the field strength in the ROI while avoiding skin intersections Copyright (c) 2024 SimNIBS developers. Licensed under the GPL v3. ''' import os from simnibs import opt_struct # Initialize structure tms_opt = opt_struct.TmsFlexOptimization() # Subject folder tms_opt.subpath = 'm2m_ernie' # Select output folder tms_opt.path_optimization = 'tms_optimization/' # Select the coil model tms_opt.fnamecoil = os.path.join('Drakaki_BrainStim_2022', 'MagVenture_MCF-B65.ccd') # Desired distance from the coil to the head in [mm] # (standard: 4 mm, as rough estimate of the hair thickness) tms_opt.distance = 0. # only perform local search (faster, usually sufficient for non-flexible coils) tms_opt.run_global_optimization = False # Select ROI in which electric field will be evaluated roi = tms_opt.add_region_of_interest() # Define a ROI on the central gray matter surface(s) roi.method = 'surface' roi.surface_type = 'central' # Center of spherical ROI in subject space (in mm) roi.roi_sphere_center_space = 'subject' roi.roi_sphere_center = [-29.90, 1.29, 72.47] # Radius of spherical ROI (in mm) roi.roi_sphere_radius = 30 # Set optimization method and parameters: 'emag' maximizes electric field strength in ROI tms_opt.method = 'emag' opt_pos=tms_opt.run()
MATLAB
% % Basic example demonstrating optimization of the electric field strength % in region of interest for a flat figure-8 coil % % The coil center will be placed to maximize the field strength in the ROI % while avoiding skin intersections % % Copyright (c) 2024 SimNIBS developers. Licensed under the GPL v3. % Initialize structure tms_opt = opt_struct('TmsFlexOptimization'); % Subject folder tms_opt.subpath = 'm2m_ernie'; % Select output folder tms_opt.path_optimization = 'tms_optimization/'; % Select the coil model tms_opt.fnamecoil = fullfile('Drakaki_BrainStim_2022', 'MagVenture_MCF-B65.ccd'); % Desired distance from the coil to the head in [mm] % (standard: 4 mm, as rough estimate of the hair thickness) tms_opt.distance = 0.0; % only perform local search (faster, usually sufficient for non-flexible coils) tms_opt.run_global_optimization = false; % Select ROI in which electric field will be evaluated tms_opt.roi = opt_struct('RegionOfInterest'); % Define a ROI on the central gray matter surface(s) tms_opt.roi.method = 'surface'; tms_opt.roi.surface_type = 'central'; % Center of spherical ROI in subject space (in mm) tms_opt.roi.roi_sphere_center_space = 'subject'; tms_opt.roi.roi_sphere_center = [-29.90, 1.29, 72.47]; % Radius of spherical ROI (in mm) tms_opt.roi.roi_sphere_radius = 30; % Set optimization method and parameters: 'emag' maximizes electric field strength in ROI tms_opt.method = 'emag'; run_simnibs(tms_opt)
The optimization will create the Gmsh output file ernie_MagVenture_MCF-B65_emag-optimization_surface_mesh.msh
with the ROI, the optimized field and the coil position:
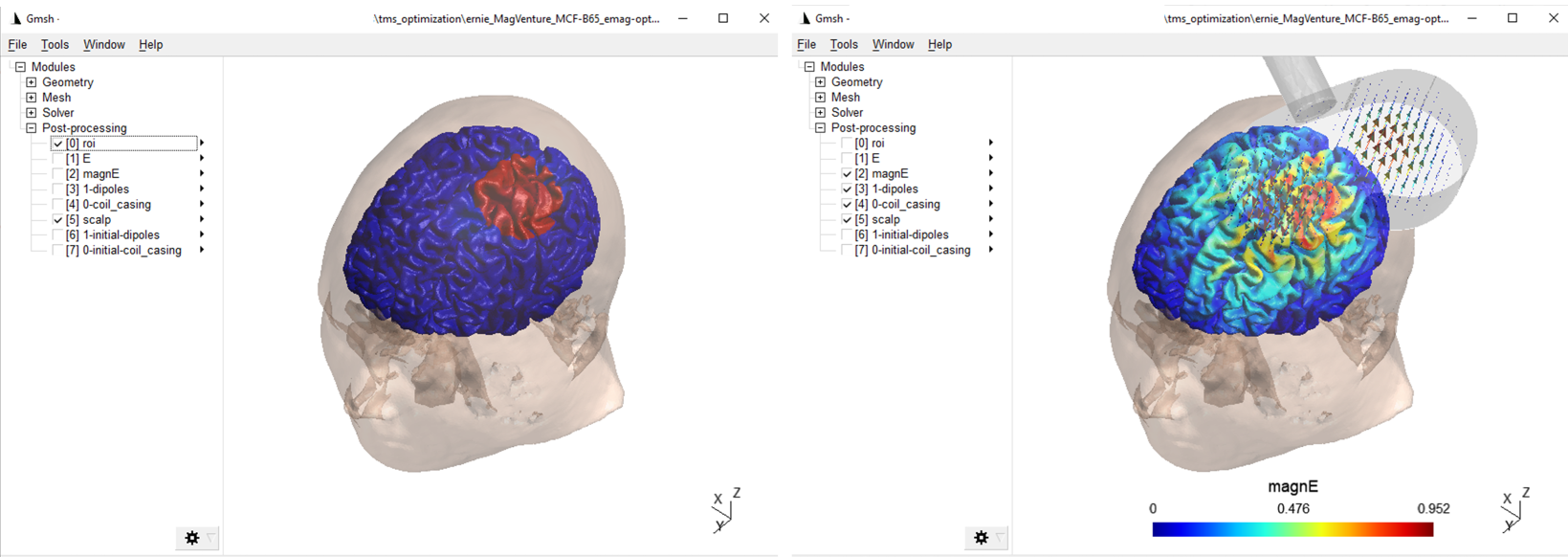
Example 2: Optimizing the position and shape of a flexible coil¶
The following example optimizes the postion of a Brainsway H1 coil so that it fits smoothly on the head. The starting position of the coil center (as defined by the company) is a scalp position above the left DLPFC. In this specific case, the starting coil position is not orthogonal to the local skin orientation underneath the coil center, but is tilted. Thus, the coil position cannot be defined by a center position and a second position indicating the y-direction. Therefore, the coil position has been defined as 4x4 matrix in MNI space. In addition, the translation and rotation ranges that control the extent of position changes to “sensible” ranges for this situation, instead of using the predefined standard ranges. This is recommended for flexible large coils to ensure good results.
Python
''' Distance optimization for a Brainsway H1 coil The coil center will be placed as close as possible (both in terms of position and orientation) to the defined position while avoiding skin intersections Copyright (c) 2024 SimNIBS developers. Licensed under the GPL v3. ''' import os from simnibs import opt_struct, mni2subject_coilpos # Initialize structure for optimization tms_opt = opt_struct.TmsFlexOptimization() # Subject folder tms_opt.subpath = 'm2m_ernie' # Select output folder tms_opt.path_optimization = 'tms_optimization_H1/' # Select the coil model tms_opt.fnamecoil = os.path.join('flexible_coils', 'Brainsway_H1.tcd') # Desired distance from the coil to the head in [mm] # (standard: 4 mm, as rough estimate of the hair thickness) tms_opt.distance = 0 # Select target position (here: via matsimnibs in MNI space) matsimnibs_MNI = [[ -0.8660, 0. , 0.5 , -44.], [ 0. , 1. , 0. , 40.], [ -0.5 , 0. , -0.8660, 59.], [ 0. , 0. , 0. , 1.]] pos = tms_opt.add_position() pos.matsimnibs = mni2subject_coilpos(matsimnibs_MNI, tms_opt.subpath, tms_opt.distance) # Set optimization method and parameters: 'distance' minimizes distance to the skin tms_opt.method = 'distance' # Note: translations and rotations are defined in the "coil coordinate system": # origin in the initial coil position, # z-axis pointing orthogonally into the head surface, # y-axis defined by pos.pos_ydir (set arbitrarily when using auto init) # # translations relative to initial position in [mm] tms_opt.global_translation_ranges = [[-20, 20], [-20, 20], [-30, 30]] # rotations of +/- degrees around all three axis tms_opt.global_rotation_ranges = [[-30, 30], [-10,10], [-5, 5]] opt_pos=tms_opt.run()
MATLAB
% % Distance optimization for a Brainsway H1 coil % % The coil center will be placed as close as possible (both in terms of % position and orientation) to the defined position while avoiding skin % intersections % % Copyright (c) 2024 SimNIBS developers. Licensed under the GPL v3. % Initialize structure for optimization tms_opt = opt_struct('TmsFlexOptimization'); % Subject folder tms_opt.subpath = 'm2m_ernie'; % Select output folder tms_opt.path_optimization = 'tms_optimization_H1/'; % Select the coil model tms_opt.fnamecoil = fullfile('flexible_coils', 'Brainsway_H1.tcd'); % Desired distance from the coil to the head in [mm] % (standard: 4 mm, as rough estimate of the hair thickness) tms_opt.distance = 0; % Select target position % (here: via coil center and coil axis orientations in MNI space) center_MNI = [-44., 40., 59.]; ydir_MNI = [0., 1., 0.]; zdir_MNI = [0.5, 0., -0.8660]; tms_opt.pos = sim_struct('POSITION'); tms_opt.pos.matsimnibs = mni2subject_coilpos(center_MNI, ydir_MNI, zdir_MNI, ... tms_opt.subpath, tms_opt.distance); % Set optimization method and parameters: 'distance' minimizes distance to the skin tms_opt.method = 'distance'; % Note: translations and rotations are defined in the "coil coordinate system": % origin in the initial coil position, % z-axis pointing orthogonally into the head surface, % y-axis defined by pos.pos_ydir (set arbitrarily when using auto init) % % translations relative to initial position in [mm] tms_opt.global_translation_ranges = {[-20, 20], [-20, 20], [-30, 30]}; % rotations of +/- degrees around all three axis tms_opt.global_rotation_ranges = {[-30, 30], [-10,10], [-5, 5]}; run_simnibs(tms_opt)
The optimization will create the Gmsh output file ernie_Brainsway_H1_distance-optimization_head_mesh.msh
with the optimized field and coil position:
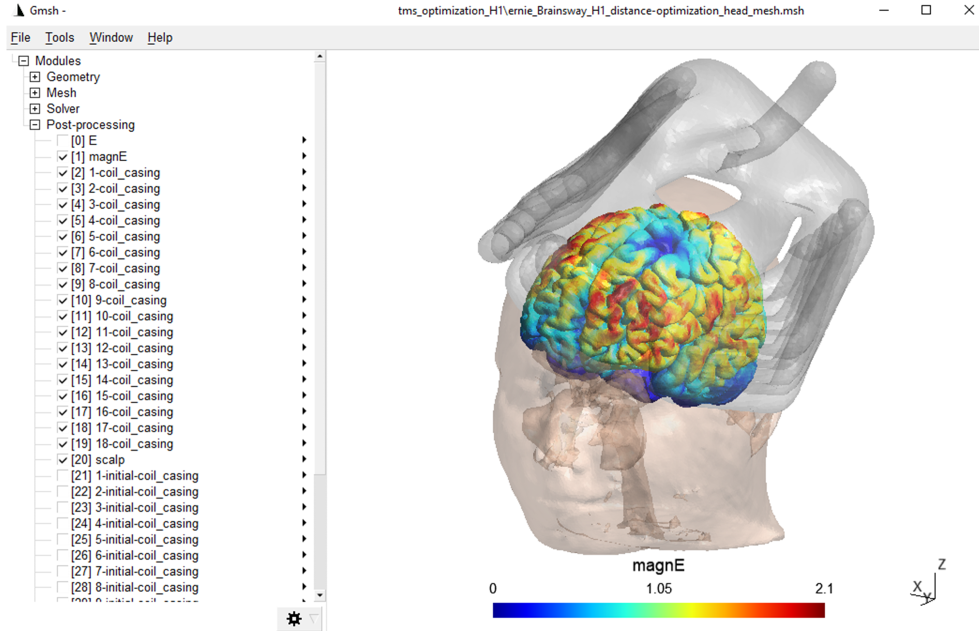
Notes¶
Setting a suited starting position is recommended for flexible coils also when maximizing the electric field magnitude in a ROI.
When maximizing the electric field magnitude in a ROI, the general-purpose TMS optimization uses the MKL Pardiso direct solver for accelerating the simulations. The SimNIBS standard FEM solver can be chosen optionally to reduce memory consumption, but will also substantially slow down the optimization.
32GB main memory are recommended, even thougth some optimizations will run with 16GB main memory.
A combination of global and local search with settings that balance efficiency with robustness in finding a good solution is used as standard. For non-flexible coils, disabling global search (setting parameter run_global_optimization to False) will work fine for most situations. In case a more exhaustive optimization is desired, we suggest to set the “locally_biased” argument of the DIRECT solver to False.
Please see TmsFlexOptimization for a description of the option settings, and RegionOfInterest for a description of the region-of-interest settings.